7 Skills to Master Before Taking the DEVASC Exam
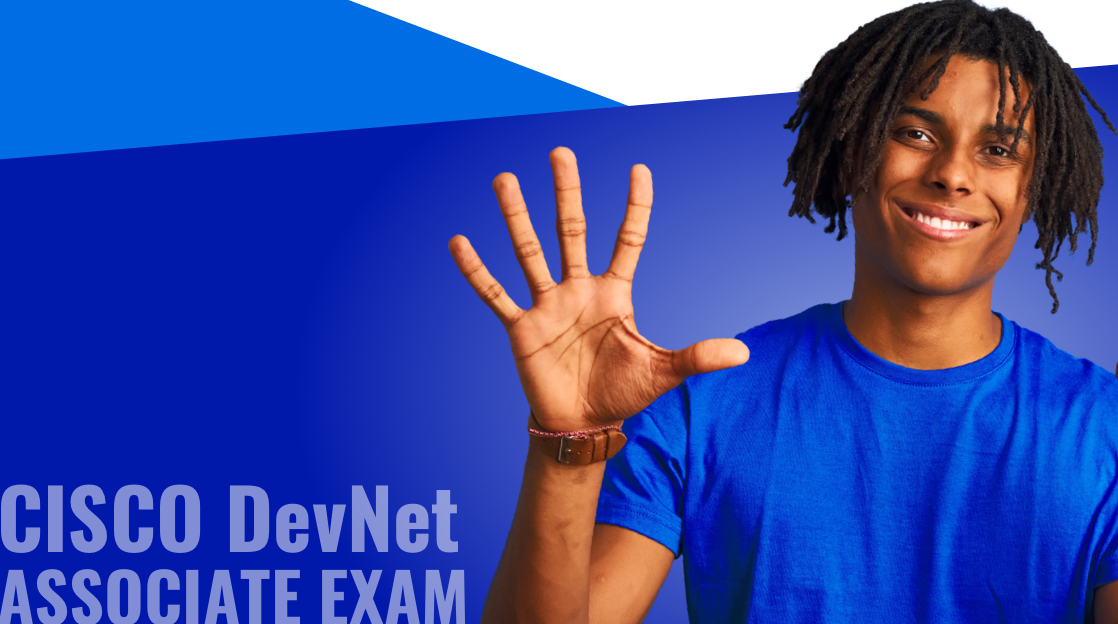
Quick Definition: The Cisco DevNet Associate certification (DEVASC 200-901) validates your knowledge in software development, APIs, Cisco platforms, application security, and infrastructure automation. As an entry-level developer certification, it shows you can blend software development skills with Cisco networking technologies.
Are you looking to boost your potential in your network career? The Cisco DevNet Associate certification (DEVASC 200-901) is a perfect starting point if you are coming from a traditional networking background and are moving towards network automation and programmability. With the right skills under your belt, you’ll be well on your way to becoming a hybrid network developer/engineer whom companies are desperately searching for.
The DEVASC exam starts off by throwing you into the fundamentals of network automation, Python coding, and APIs. If you already have the basics like your CCNA under your belt, coding may be difficult to wrap your head around if you haven't prepped for it.
You will struggle with this exam if you don't have the right skills, so here's what you need to know before you tackle this cert.
Master Python Basics
Learning Python is a heavy requirement for the DEVASC exam. You don't have to be a coding veteran, but you should know your way around loops, functions, and classes, at the very least. These are going to be your foundational building blocks when you’re working with Cisco APIs.
The exam will test your ability to read and understand Python code snippets and write scripts that use Python libraries, such as requests for API calls and Cisco SDKs, for automation tasks.
You'll need to get hands-on with Python, not just read about it. Python works so well for network automation because it's easy to read and has great libraries for working with APIs. A great practice step is to write small scripts that solve real problems, even if they are not network-related at first. You’ll want to learn how your scripts run and get familiar with coding the basics.
After you’ve made a few scripts, you can start experimenting with networking basics like pulling device info from an API or configuring network settings from a script. Practicing with a real code editor and executing your code is going to make the concepts that you’re learning about stick around well after exam day. These are skills that you can use everywhere during your working hours (and at home).
Get Hands-On with REST APIs
The DEVASC exam goes deep into REST APIs. It's going to test your understanding of syntax, authentication, and how to troubleshoot when things go wrong. You'll get some questions where you drag-and-drop code blocks, others where you’ll interpret API calls, and even some where you’ll have to pick the right methods for different jobs.
CRUD is an acronym for data actions with applications. (Create, Read, Update, Delete) You need to know how HTTP methods work for CRUD operations:
POST creates brand new resources (Create)
GET grabs data from an API endpoint (Read)
PUT updates or replaces what's already there (Update)
DELETE removes resources you don't need anymore (Delete)
Make sure that you get familiar with query parameters that filter results (like ?status=active) and path variables that point to specific resources (like /devices/{device_id}). You'll also need to understand status codes like 200 OK or 404 Not Found, and how to handle response data properly.
Python's requests library makes it easy to work with APIs. There are public APIs that you can practice with before you even start with Cisco. This will help you nail the basics in general before you focus on APIs in the Cisco context.
The exam will test how well you understand synchronous calls (calls that block other calls that wait for a response) versus asynchronous (async) calls (calls that return a 202 Accepted status with a task ID and don’t block other calls while they are busy).
With Cisco DNA Center, you'll often start a task that runs for a long time, and then poll a separate endpoint to check the task status. Make sure that you read and can understand API docs and implement both types of calls (patterns) correctly.
Spend some time with JSONPlaceholder or HTTPBin to practice the basics, then move to Cisco's DevNet sandboxes when you're ready for the real thing.
Learn How to Authenticate API Calls
Authentication is very important for API security, and the DEVASC exam asks a lot of questions about it. You need to understand multiple authentication methods and how to use them.
Get to know these key authentication approaches:
OAuth 2.0 flows give you secure tokens for accessing resources. Learn about the two main types: client credentials flow for machine-to-machine communication and authorization code flow for when users need to give permission to an app.
API key authentication is quite straightforward: you’ll pass a pre-generated key in your request headers (usually as X-API-Key or Authorization). This tells the API who you are, and what you're allowed to do.
Basic authentication uses a username and password encoded in Base64 in the Authorization header. It can be secured when you use it with HTTPS/TLS encryption. In recent APIs, token-based methods have mostly been replaced because of their limitations. The exam might test whether you understand both methods and when you might use one or the other.
JSON Web Tokens (JWTs) contain user info and claims (permissions) in a format that is encoded. They're used for something called stateless authentication, usually between services since they don't need session storage on the server.
Where it gets complicated is that each Cisco platform handles authentication slightly differently. Meraki wants an API key in the header, but DNA Center uses OAuth 2.0 to get tokens. If you know these differences, it helps you tackle exam questions about choosing the right authentication method for different scenarios in your questions.
You’ll need to practice building request headers for different APIs and also troubleshoot authentication failures by reading error messages. Again, it's not a bad idea to start with simple API key auth on public APIs before you move to more complicated Cisco platform authentication methods.
Work with Cisco-Specific APIs
The DEVASC exam covers multiple Cisco platforms and their APIs. Each one has its own setup and set of capabilities that you'll need to understand for the exam.
Cisco DNA Center provides intent-based networking with RESTful APIs. These APIs are organized in a hierarchy for network discovery, device management, and automation. Practice with site management and device onboarding APIs, too; they often appear in the exam.
The Meraki Dashboard gives you cloud-managed APIs for device provisioning and monitoring network health across different sites. Focus on getting network details and device status using these APIs.
Webex Teams APIs let you create rooms, send messages, and manage participants programmatically via scripts and apps. Try building small scripts that automate these functions and figure out what works, and what doesn’t. There is a lot of API documentation available that you can use as a solid reference guide.
SD-WAN (Viptela) APIs are used to manage software-defined WAN environments. They use template deployment and policy management across distributed networks, which is their main focus.
One of the best ways to learn these APIs is by using Cisco's DevNet sandboxes. These are free environments that give you access to real (and virtual) devices for testing your API calls without worrying about breaking production systems. There are ‘Always on’ devices that don’t require a reservation before using them, and ‘Reservation Sandboxes’ that you’ll need to book in advance before you can access them.
The exam might ask you to identify the right API endpoint for a specific task or interpret response data to pull out important information. Spend time with each platform's API documentation along with your hands-on practice. Reading and doing at the same time is a great combo, and it will help you prepare for the platform-specific questions on the exam.
Get Familiar with JSON & Data Formatting
JSON is the language that APIs speak, and you'll need to work with it comfortably for the DEVASC exam. The test will check if you can parse JSON responses and get the data you need.
Here are some Python skills for handling JSON:
Using json.loads()
Turns JSON strings into Python dictionaries and lists. This lets you access values using Python's key value syntax, which is valuable when you need to access specific information in your data:
import json
json_string = '{"name": "Router1", "status": "active"}'
data = json.loads(json_string)
print(data['name'])
#Result: Router 1
With json.dumps()
You can convert Python objects back into JSON format when you need to send data in API requests:
python_data = {"name": "Router1", "status": "active"}
json_string = json.dumps(python_data, indent=4)
print(json_string)
#Result
{
"name": "Router1",
"status": "active"
}
Cisco APIs sometimes return deeply nested JSON structures. You'll need to dig through quite a few levels to find what you want:
nested_json = {"device": {"id": 1, "details": {"name": "Router1", "status": "active"}}}
print(nested_json['device']['details']['name'])
# Output: Router1
Python has many tools you can use to parse JSON. Try to get familiar with different tools that make JSON handling a lot easier:
jsonpath-ng for complex queries in deeply nested format structures.
pandas turns massive JSON datasets into DataFrames for easier analysis.
pprint makes JSON more readable during development.
As a self-test, try writing a script that pulls data from a Cisco API, extracts certain fields from the JSON response, and then formats the output. Compare how you'd do this with standard dictionary handling against using jsonpath-ng to see which works better, and which you prefer.
The exam could show you a JSON response and ask you to write code that pulls out certain values or transforms the data. It’s quite straightforward, but you have to practice to nail the syntax. If you’re comfortable with basic Python techniques, you will save yourself time on exam day.
Understand Docker, Terraform & Automation Tools
Infrastructure as Code is a big part of modern networking, and the DEVASC exam tests your knowledge of common tools like Docker and Terraform. You don't need to be an expert for the exam, but you should know the basics and how they work in Cisco environments.
For Docker, focus on these areas:
Learn all about container basics. Learn why containers are different from virtual machines, how isolation works, and what image layers are.
For the exam, focus on the basics of container networking - especially how bridge networks isolate containers, and when to use host networking for performance. Learning about these scenarios will help you on exam day.
Learn commands for running and managing containers—starting, stopping, checking logs, and running commands inside containers.
Practice building custom images with Dockerfiles that include the right base images and dependencies for network tools.
For Terraform, you'll need to understand:
HashiCorp Configuration Language (HCL) basics. This is the language for defining infrastructure.
The terraform plan and apply workflow. Learn how to review and apply infrastructure changes.
Basic state concepts. Learn what state files do and why they matter.
The exam generally focuses on Terraform's use in network automation, so concentrate on that and familiarize yourself with it.
When you practice, try using Cisco DevNet Sandboxes so that you can mock deploy these tools in realistic scenarios. For Docker, try experimenting with different network modes and build images with network tools. For Terraform, create configurations that automate Cisco infrastructure, such as ACI policies or Meraki devices.
Remember, the exam will ask about container networking, environment variables, and infrastructure automation with Cisco platforms. You should have some practical, hands-on experience with these tools because it will make these questions much easier to handle.
Exam Strategy and Time Management
The DEVASC exam gives you 120 minutes to complete 90-110 questions.
Here's how to manage your time effectively:
Do a quick first pass through all of the questions, and quickly answer the ones you're confident about, and flag the rest for review.
For drag-and-drop coding questions, look for clear starting points (like import statements) and ending points (like return statements) to help arrange the code logically.
If you're stuck on a question for more than 2 minutes, flag it and move on—you can come back to it if there is time.
Keep an eye out for questions that give you hints for other questions—sometimes later questions contain information that helps with earlier ones.
Hands-on questions are the trickiest, but also the most valuable for passing. If coding is not your strongest skill, then dedicate some extra practice time for it. Review the drag-and-drop and code completion exercises when you use Cisco's DevNet Learning Labs.
Study Real Exam Questions & Drag-and-Drop Syntax
The DEVASC exam has different question types, like drag-and-drop coding questions that test your knowledge of arranging code snippets in the right order. These questions check whether you can write and understand code in Cisco environments, not just theoretical concepts.
Look at how Cisco structures API calls in their documentation. Learn their patterns for handling authentication, formatting requests, and processing responses. The exam will test if you can recognize and use these patterns properly.
Get practice with common libraries used with Cisco APIs:
requests for making HTTP API calls to Cisco platforms.
netmiko for CLI automation for network devices.
ncclient for NETCONF interactions with supported devices.
Cisco's own SDK libraries for platforms like Meraki, DNA Center, and ACI.
Learn how Cisco recommends handling API errors, including retry mechanisms and error parsing. Practice arranging code blocks that implement authentication steps for different Cisco platforms, such as OAuth flows for DNA Center or API key usage for Meraki.
Don't get stuck on generic Python exercises. Find practice tests with Cisco-related coding questions that match the syntax patterns on the exam. Cisco's DevNet Learning Labs are perfect for this since they use the same conventions and patterns that you'll see on the test.
Remember that the exam is timed, so you need to recognize correct code patterns quickly. Getting familiar with Cisco's API documentation and syntax will save you valuable time during the test.
Conclusion
The DEVASC 200-901 exam is not the kind of exam that you can prepare for without actually doing the practical work. The seven skills that we covered are exactly what Cisco tests for, so learn as much as you can about them.
When you add these skills with hands-on practice in DevNet sandboxes, you'll also develop real coding skills, which is what makes this cert valuable. Spend time mastering these concepts, and you’ll turn into the hybrid network developer/engineer that companies are looking for.
Check out our DEVASC 200-901 course to get the info you need to prepare.
delivered to your inbox.
By submitting this form you agree to receive marketing emails from CBT Nuggets and that you have read, understood and are able to consent to our privacy policy.