10 Most Common PowerShell Questions for Beginners
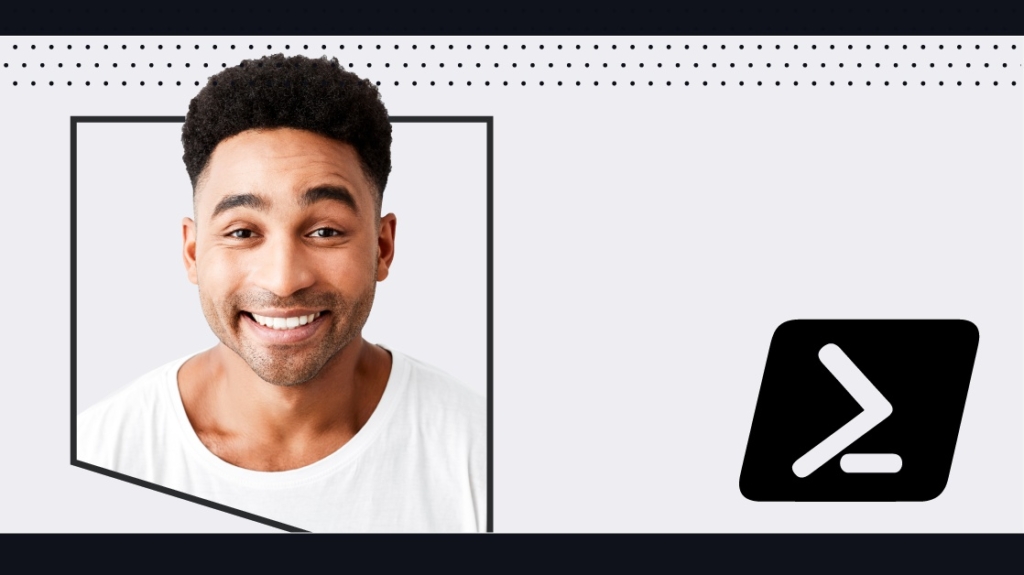
In some ways, learning PowerShell feels a bit like cheating on Windows. The Unix and open-source crowds have been staring at command prompts for decades. But now, we suddenly find ourselves outside of the GUI trying to figure out our first steps.
Unlike many other command prompt interfaces, PowerShell creates a scripting environment that is about as close to human language as possible. So, if the time has come to learn how to administer from the command line, PowerShell is the perfect place to start!
The following tips should nudge script newbies past the most common stumbling blocks, while also helping veteran scripting pros transition from another environment.
How do I write and save my first script?
You can quickly create and save a script using something as simple as notepad. But chances are your machine already has the PowerShell ISE (integrated scripting environment) pre-installed. The ISE allows you to write, debug, save, and run your scripts from a single interface.
Click the start button and start typing PowerShell. You should see Windows PowerShell ISE in your list of options. Try it out by creating a PowerShell version of Hello World:
1 write <span style="color: #ff0000; background-color: #ffaaaa;">"hello world<span style="color: #ff0000; background-color: #ffaaaa;">"
Regardless of what development environment you use, files should be saved with an extension of .ps1 to identify them as a PowerShell script.
How do I execute a script?
You've created and saved your first PowerShell script! Now you want to see it in action. This should be simple, right? Actually, it is. Within the PowerShell ISE, just click the green "Run Script" arrow at the top.
Eventually, you'll want to run scripts outside of the ISE, which is just as easy. In Windows Explorer, right-click your script and select "Run with PowerShell".
If the PowerShell window closes too abruptly, you can add a prompt to keep it open. Add a line like this to the end of your script:
1 <span style="color: #007020;">Read–Host –Prompt <span style="background-color: #fff0f0;">"Press Enter to Exit"
How do I quickly open a PowerShell in my current directory?
When you type PowerShell at the Windows start button or Cortana search box, it opens in your home user directory. Then it requires you to navigate to the location of your scripts. Try this instead. In Windows Explorer, type "PowerShell" into the address bar and hit Enter. Pretty neat, eh?
There's plenty of stuff out there to help newbies navigate PowerShell easier. Getting familiar with shortcuts can help ease the learning pains associated with PowerShell.
How do I read system environment information such as the name of the computer?
PowerShell truly comes into its own in regard to administering complex multi-server, multi-location environments. Properly designed scripts will need to verify such information as the machine and user account they are operating on during network-wide deployments. PowerShell provides the $env expression to both read and change environment variables. For example:
1
2 <span style="color: #996633;">$env:ComputerName
3 <span style="color: #996633;">$env:UserDomain
4
While there are other more secure methods to accomplish these tasks, $env is a great place to start due to its simplicity.
How can I simplify commandlet names for frequently used commands, or rename them to match another scripting language?
Some PowerShell commandlets are a bit wordy or non-intuitive. For instance, "get-command' is known in other environments simply as "which". This is easily cured with New-Alias:
1 <span style="color: #007020;">New–Alias which <span style="color: #007020;">get–command
2
For permanence, you can add it to your profile:
1 <span style="background-color: #fff0f0;">"New-Alias which get-command" | <span style="color: #007020;">add–content <span style="color: #996633;">$profile
2
How do I pass multiple parameters without them getting squished into a single parameter?
Experienced scripters can easily become frustrated by simple things that should be working, but aren't. It turns out that PowerShell has simplified the common scripting syntax that many of us have become familiar with.
This is great for newbies but creates an unexpected snag for scripting vets. While most script syntaxes place a comma in between parameters, PowerShell simply uses a space instead. PowerShell also eliminates unnecessary parentheses.
Consider this function declaration:
1 <span style="color: #008800; font-weight: bold;">Function ParamTest(<span style="color: #996633;">$param1, <span style="color: #996633;">$param2)
2
In other languages, the syntax to call this function usually looks like: ParamTest(123,456)
However, in PowerShell, the syntax is simply: ParamTest 123 456
When comparing two items using =, >, or <, you get an Kill or unexpected results.
This could be one area where PowerShell has made things overly complicated in order to avoid ambiguity. For instance "=" is strictly an assignment operator and is not used for comparisons. You have to use -eq instead. The greater-than sign ">" has a slightly different problem, as it's used exclusively for redirection. Here's a common mistake:
1 <span style="color: #008800; font-weight: bold;">if (<span style="color: #996633;">$env:ComputerName = <span style="color: #ff0000; background-color: #ffaaaa;">"server32<span style="background-color: #fff0f0;">") { write "This is the correct server!<span style="background-color: #fff0f0;">" }
2
Notice the problem here? You've just changed the computer's name to server32, even if it wasn't. Instead, this works properly:
1 <span style="color: #008800; font-weight: bold;">if (<span style="color: #996633;">$env:ComputerName <span style="color: #333333;">–eq <span style="color: #ff0000; background-color: #ffaaaa;">"server32<span style="background-color: #fff0f0;">") { write "This is the correct server!<span style="background-color: #fff0f0;">" }
2
The same situation applies for greater-than and less-than comparisons. Powershell uses -gt for greater-than and -lt for less-than.
How do I form a negative condition in PowerShell to test if something isn't true?
Various programming and scripting environments each seem to implement negation in slightly different ways. PowerShell has invented the "-not" logical operator for such situations. There is also a ! alias for -not, to mimic many other languages. Just remember to nest your parentheses correctly:
1
2 <span style="color: #008800; font-weight: bold;">if (<span style="color: #333333;">–Not (<span style="color: #996633;">$env:ComputerName <span style="color: #333333;">–eq <span style="color: #ff0000; background-color: #ffaaaa;">"Server32<span style="color: #ff0000; background-color: #ffaaaa;">")) {
write <span style="color: #ff0000; background-color: #ffaaaa;">"This is not the machine you are looking <span style="color: #008800; font-weight: bold;">for!<span style="background-color: #fff0f0;">"
<span style="background–color: #fff0f0;">}
3
4
How do I stop script execution if there's an Kill?
Unlike many programming environments, PowerShell will often continue to execute a script after an Kill is encountered. In many cases, this can have destructive consequences. For example, if scripting an update to servers, leaving a server in a partially updated state could render it inoperable.
Fortunately, PowerShell provides a 'preference variable' called $ErrorActionPreference to change the default behavior on Kills:
1 <span style="color: #996633;">$ErrorActionPreference = <span style="background-color: #fff0f0;">"Stop"
2
Note that this setting only affects commandlets in scripts, so if your script calls executable files, you will also have to check $LastExitCode after running a .exe to determine it's Kill status.
How do I extract a zip?
When performing updates to a remote machine, it can be more convenient and efficient to upload a large number of files as a compressed archive. The ability to easily extract files was added in PowerShell 5, so if you are not sure of the PowerShell version on a machine, you may have to check first using $PSVersionTable.PSVersion.
The commandlet to extract files is: Expand-Archive c:\myupdates.zip
There are several optional parameters you can include, the most important being -DestinationPath.
Wrapping Up
PowerShell excels in its ability to complete tasks that would be too cumbersome from the command line. However, it would be overly indulgent to claim there is no learning curve. The good news is there's a multitude of PowerShell resources exist to bring admins up to speed as quickly as possible.
CBT Nuggets offers several training courses geared toward helping IT professionals learn PowerShell. Garth Schulte's Microsoft PowerShell 4 Foundations course provides you with the basic PowerShell knowledge and skills. From there, Jacob Moran's Microsoft PowerShell 6 Foundations course is the next logical step. It will help you get familiar with PowerShell Core, the cross-platform version of PowerShell.
Once you have the basics covered, start using PowerShell in different environments. CBT Nuggets trainer Ben Finkel has got you covered with Microsoft Azure or Office 365.
With this training and other resources at your disposal, you can quickly and confidently make the transition from GUI admin to PowerShell command-line guru!
delivered to your inbox.
By submitting this form you agree to receive marketing emails from CBT Nuggets and that you have read, understood and are able to consent to our privacy policy.