What Are the Basics of Go Syntax?
The Go programming language is known for its scalability, ease of use, and low memory overhead.
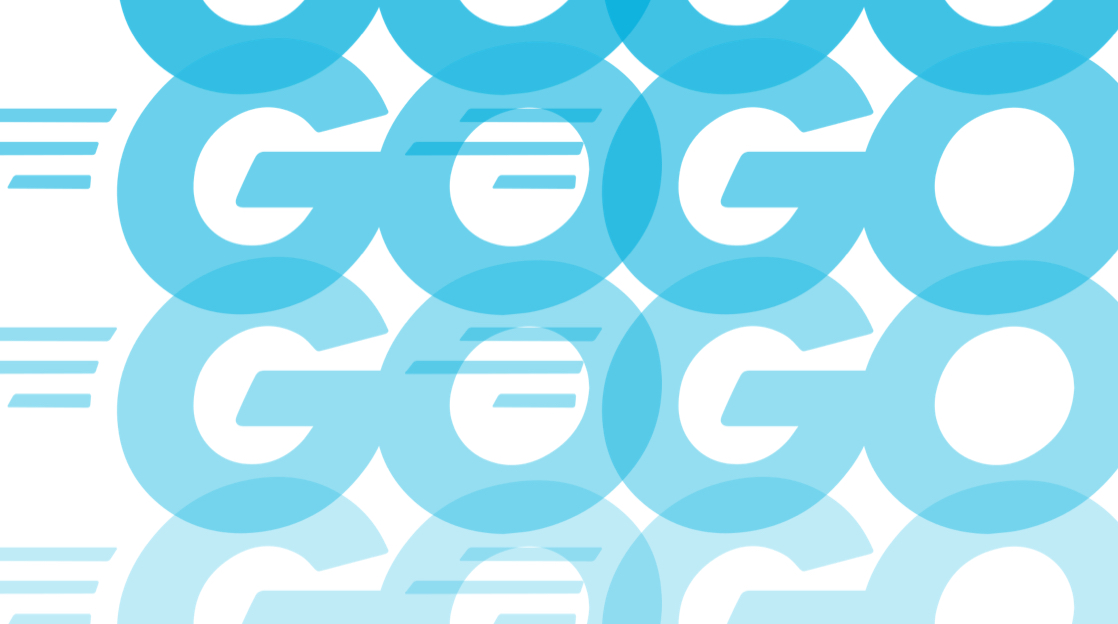
Every now and then, a programming language comes along that truly makes a splash in the IT ecosystem. In 1972, it was C. In 1995, it was Java. In 2007, it was Golang’s turn, and the Go language is still making waves today. Though it wasn't released by Google as an open source programming language until 2012, Go’s versatility, simplicity, and scalability have drawn thousands of developers to learn Go since its early days — and that journey always begins with Go syntax.
We'll cover the basics of Go syntax shortly, but first, get a better understanding of how (and why) Go came into existence. Once we've covered the reason why Go was created in the first place, we can dive into Go syntax with a better understanding of its primary use case.
The Go Programming Language: An Origin Story
By the mid-2000s, massive amounts of computational power were becoming necessary. Google’s servers were making billions of requests, and its web crawlers were trawling hundreds of millions of websites. At the time, there weren't any programming languages that could facilitate this sort of massive data analysis. This is where Golang came in.
Originally known as Golang (now just "Go"), the Go language was written almost entirely in C, which is why Go syntax is quite similar to C syntax. If you have experience writing in C, then Go syntax should be familiar to you. However, you might be surprised to learn that Go, unlike C, takes care of its own garbage collection, and memory management is handled under the hood.
The Go compiler, on the other hand, is written in a combination of Go and C. For the skeptics, here is an example of a “Hello World” programming in Go, and then the same thing in C.
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
Then run:
go run hello.go
Now, the same thing in C:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
Save the file as hello_world.c and run:
gcc hello_world.c -o hello_world
./hello_world
That is a very simple Go program. Let’s look at one that has a little more meat on it and really dissect it.
How to Create a Go Program: Go Tutorial
One thing to understand immediately about Go is that it is more than just an object-oriented programming language. So, there is no way of defining a “class” in the same way you would in C#, Java, or even Python. Instead, you’ll create structs and call that struct in a method. Let’s take a look at an example:
package main
import "fmt"
// Define a struct type
type Person struct {
name string
age int
}
// Define a method associated with the Person struct
func (p Person) introduce() {
fmt.Printf("Hello, my name is %s and I am %d years old.\n", p.name, p.age)
}
func main() {
// Create an instance of the Person struct
person := Person{
name: "Alice",
age: 30,
}
// Call the introduce method on the person instance
person.introduce()
}
Let’s go line by line and discuss what each code section is doing.
The first portion of the Go syntax will always be package declaration. Packages in Go are used to organize code and facilitate reusability.
The package declaration is always proceeded by numerous import statements. In the example of our Person struct, we are importing in the external library “fmt”. This library provides standard I/O (In/Out) procedures, such as printing outlines to the computer terminal.
Next, we’ll talk about the meat and potatoes of the program itself — the structs and methods.
Go Syntax: What is a Struct?
The next line of Go Syntax is a struct. A struct is a key/value-based entity that defines what a piece of data is supposed to look like. Notice in our Person struct, we provided an “age” and “name.” The name is of a data type string, and the age is an integer. In Go, the user must define the type of data for a given struct. The next line of code may seem a little odd because it is a method that takes a receiver.
Go Syntax: What is a Method?
The next line is a method called introduce. It takes zero parameters; however, something interesting precedes the method name. It is a receiver called (Person p). In Go, this is how developers associate methods with specific structs. This receiver allows a method to access a strut’s attributes. In this case, those attributes are p.name and p.age.
As you can see, Go syntax is a little different from object-oriented programming. In Java, for example, an entire class is created, and in it, a developer will define the data structure and associated methods.
But in Go, the process is streamlined and syntactically decouples these two processes.
The last block of code is the main() function. In this portion of the code, we are instantiating a Person class with the name Alice and giving her the age of 30. Lastly, we call the “introduce()” method. When this program is run, it will output the following:
“Hello, my name is Alice and I am 30 years old.”
Recap of Go Tutorial Basics
Go Syntax often revolves around structs and methods. We can use receivers to access the attributes of structs inside methods. Lastly, we instantiated the Person class, which allowed us to call the introduced method on the object we created.
There are hundreds of programming languages that can help you build the skillset needed to create efficiencies and improve day-to-day life. Go is certainly one of the newer ones, but there are so many more that are worth your time. Learn Python, MySQL, PowerShell, VS Code, HTML, CSS and JavaScript with a high-quality training you can watch at your own pace.
Not a CBT Nuggets subscriber? Sign up for a 7-day free trial now!
delivered to your inbox.
By submitting this form you agree to receive marketing emails from CBT Nuggets and that you have read, understood and are able to consent to our privacy policy.