How to Analyze Network State with Scrapli
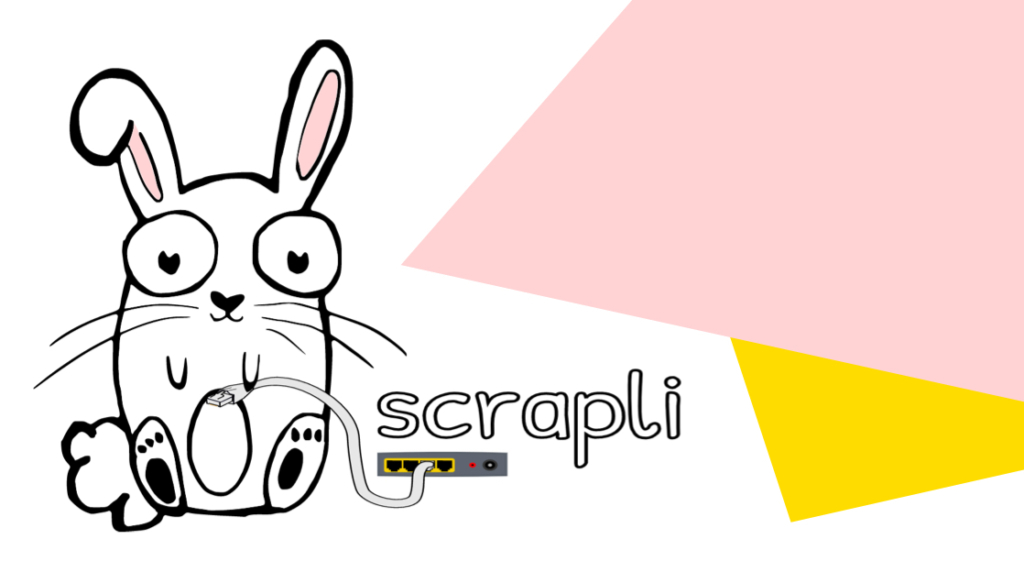
It's no secret that the duties and responsibilities of network engineers are evolving at breakneck speed. In particular network automation, which had been a curiosity at first, but now plays a pivotal role in an organization's success. The network automation market is set to hit more than 22 billion dollars by 2027. With that being said, rest assured it is not a fad technology.
To automate a network, it is necessary to have a broad understanding of the Python programming language — particularly the API known as Scrapli. In this post, we'll explain what Scrapli is, how to install it, and how to use it to analyze the state of your network.
What is Scrapli?
Scrapli is a Python library used to connect devices together, such as routers and switches. Scrapli supports several different devices such as Cisco IOSXE, IOSXR, Juniper, Arista, and more. In a nutshell, device connectivity is accomplished by creating a driver object and then sending commands to the router. We'll get to that later.
Scrapli is a portmanteau of "Scrape" and "CLI", thus Scrapli. The "scrape" portion refers to the fact it utilizes screen scraping, and "li" because it is a command line interface. (CLI) Scrapli can be used for virtually any network operation imaginable, from analyzing network state to configuring backups, rollout or network validation. Let's take a look at how to install Scrapli onto your computer.
How to Install Scrapli
Generally, Scrapli will be installed as a PIP install. For this post we will assume you have Python 3 installed. PIP stand for Python Installation Package. It is a dependency manager for the Python programming language. PIP is a critical component of Python, so it has been included in every Python installation since version 3.4. After all, pip is not only for Scrapli. It is for virtually any other API you would like to use for Python such as pandas, flask, and more.
To verify you have pip, simply open up your terminal or command prompt and type pip. If all goes well, then it should display a list of commands. Next type pip install scrapli and hit enter. Wait a few minutes for the installation to complete and voilà!
How to Analyze Network State with Scrapli
Now that we know what Scrapli is and how to install it, it is time to get our hands dirty. Just to make sure we are all on the same page, let's define "network state". For this post, the state of the network is either "up" or "down". Naturally, the desired state is "Up", meaning all devices are in an operational state. "Down" is naturally the opposite.
Network state can be determined by analyzing the routers and switches that the network data flows through. This data can be processed via Scrapli. So let's get started.
How to Import Scrapli Into Python
First, open up Visual Studio code and create a new Python file. Call it example1.py. Remember, all python files end with the .py file extension. Next we need to import scrapli into our project. So type:
MY_DEVICE = {
"host": "sandbox-iosxe-latest-1.cisco.com",
"auth_username": "developer",
"auth_password": "C1sco12345",
"auth_strict_key": False
}
What we have here are keys that Scrapli is expecting. The host is the device that will be connected to, i.e the Cisco example on DevNet. The username and password are the ones for the particular device being used. Lastly auth_strict_key is determining whether or not the keys used will be verified—it is true by default.
How to Create a Cisco Sandbox Device
Lucky for us, Cisco provides a great sandbox for us to experiment in. Go to the Cisco Development Lab and click Get started with Sandbox. Login however you like. I prefer to use my GitHub account. After logging in, search in the top left hand corner for Xe. Click on the link that says IOS XE on CSR. Use the one that says "Always On." By now, your screen should looking something like this:
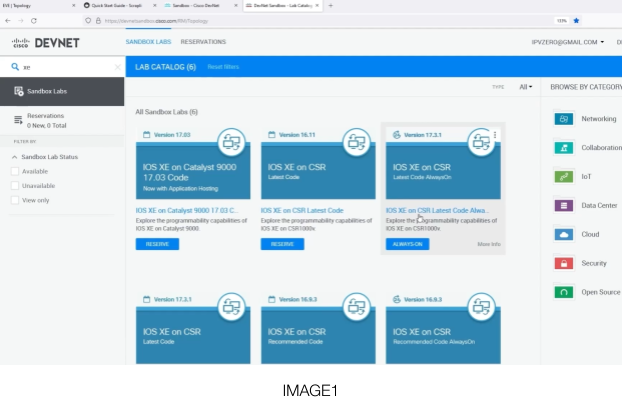
By using this host we will be able to connect without a VPN connection. Once you click on that link scroll down and find the Hostname, username, and password. Write those down, because we are going to need them. It will look just like the picture below:
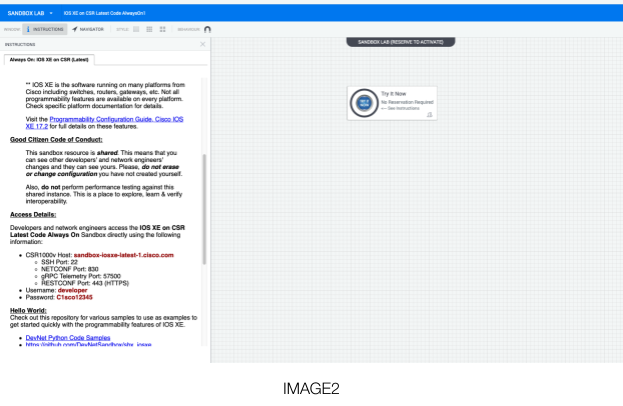
Representing Hosts In Python
Next, we need to represent our device in dictionary format. Others may call this JSON format, or key value format. A dictionary is a key value pair that the computer is able to insert and retrieve from very quickly. It is generally O(1) time. In other words, the beauty of this data structure is that it is extremely easy to insert, delete, and fetch from…assuming you provide the key.
MY_DEVICE = {
"host": "sandbox-iosxe-latest-1.cisco.com",
"auth_username": "developer",
"auth_password": "C1sco12345",
"auth_strict_key": False
}
What we have here are keys that Scrapli is expecting. The host is the device that will be connected to, i.e the Cisco example on DevNet. The username and password are the ones for the particular device being used. Lastly auth_strict_key is determining whether or not the keys used will be verified—it is true by default.
How to Connect to Devices Using Python
You have imported the required driver, and represented a device using our dictionary notation. However, that was just teeing everything up, now we will write the code to execute the actual connection.
Because Python is (more or less) an object-oriented programming language, we will instantiate an object that will drive the connection to our DevNet router. Please write the following code beneath the import statement:
conn = IOSXEDriver(**My_Device)
We now have an object to work with. The object's type is IOSXEDriver, which means we can use all the methods associated with that class. If Object Oriented Programming is brand new to you, it would be worthwhile to check out this great course.
The double asterisk is intentional, and it is how we are unpacking the dictionary for use by our driver. However, this is a fairly naive way of accomplishing what we want. Python provides context management to look after this object—that way we don't have to close and open the connection manually. Replace that text with this:
with IOSXEDriver(**MY_DEVICE) as conn:
Using a with statement is considered best practice. We have instantiated the object using the with keyword instead of doing it the other way.
Building and Reading a Scrapli Router Response
Now that the object is created, it is time to do something with it. This is where the Scrapli magic really happens. In the same vein as creating an object for the IOSXEDriver, we will instantiate an object for the response. In this context, a response is what the router returns to us when we make a specific query. A query will look like this:
response = conn.send_command("show ip interface brief")
So far, your code should look like this. Do NOT forget about the indentations, it is how Python reads code.
from scrapli.driver.core import IOSXEDriver.
MY_DEVICE = {
"host": "sandbox-iosxe-latest-1.cisco.com",
"auth_username": "developer",
"auth_password": "C1sco12345",
"auth_strict_key": False
}
With IOSXEDriver(**MY_DEVICE) as conn:
response = conn.send_command("show ip interface brief").
print(response.result)
The last portion of the code is actually printing the result to the console.
Executing a Python Script
Executing a Python script is similar to executing a shell script. Simply go to the directory that the file is located in and type python3 and then the name of the script. So in our case, it would be:
python3 example1.py
The picture below is an example of what it ought to look like.

Once that command is executed, it will send a request to the Sandbox router we tapped into on Cisco DevNet. It will request the state of each device on the network. The result will look like the picture below:
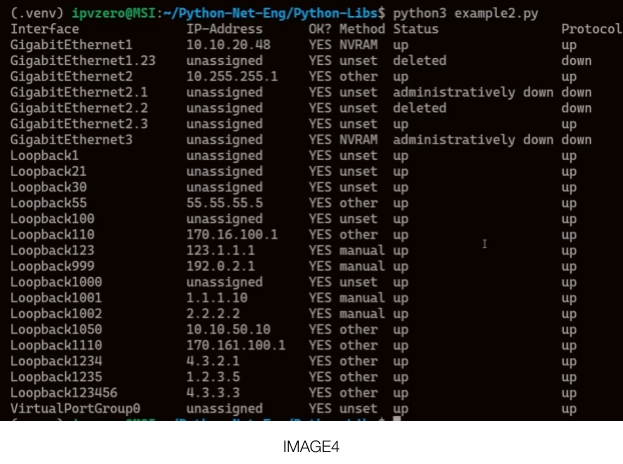
It is truly amazing that we were able to retrieve this information in less than ten lines of code. From here, it would be easy to parse this information and send out alerts via email, generate reports on the state of the network, and countless other operations. Notice the fifth column from the left is called "Status". The network is healthy, because they all say "up" or are administratively down.
Final Thoughts
Scrapli is a versatile tool that every network engineer needs to familiarize themselves with. It is also surprisingly easy to use. In fact, most people will probably have more difficulty actually learning Python than learning how to use the API itself. I would encourage any burgeoning network engineer to continue exploring different and innovative ways to query their network with Scrapli.
All of this information and more can be found in this CBT Nuggets Network Automation course. In this post, we defined what Scrapli is, how to install it, and a plausible use case. Happy coding!
delivered to your inbox.
By submitting this form you agree to receive marketing emails from CBT Nuggets and that you have read, understood and are able to consent to our privacy policy.