How to Write a Simple Ping Test with PowerShell
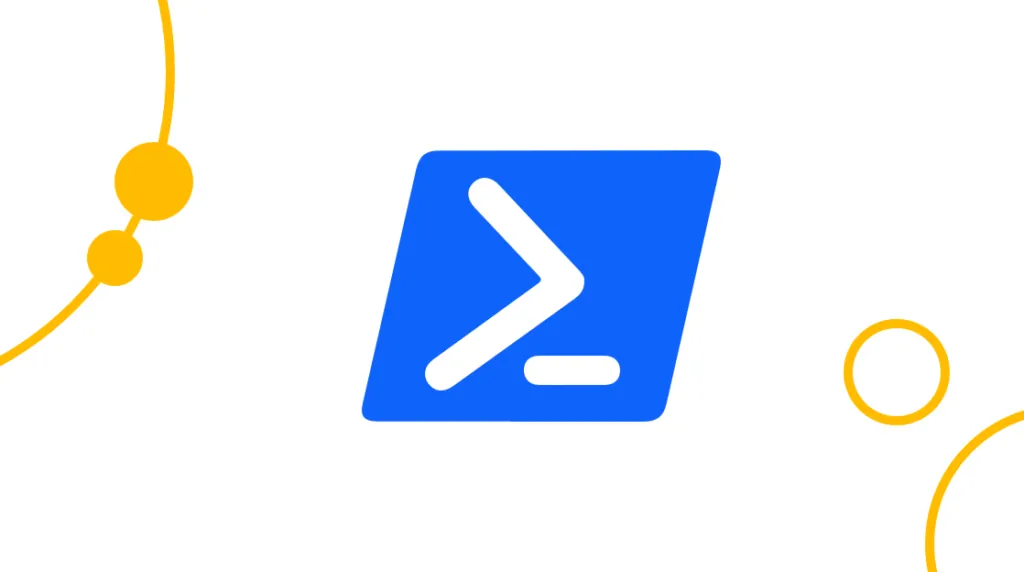
PowerShell is a very under-rated tool. This is despite the fact it has grown to be a powerful shell environment and strong scripting language. PowerShell can be used for a wide variety of IT tasks in every type of environment. One of those IT tasks is diagnosing why devices aren't connecting to a network or troubleshooting network issues. In these cases, ICMP packets are the tool of choice for IT pros.
Traditionally, the simple ping application in command prompt in Windows is the tool of choice for these types of tests. Ping is an easy way to shoot an ICMP packet to IP addresses on a network to see if there is a response or not. There is so much more information we can get from pinging a device, though. For instance, we could get the latency time for a response or the number of hops a packet needs to take to get to a device.
Today, we are going to discuss how to ping a device with PowerShell — and how to use Pester to create tests.
What is Pester?
Pester is a framework to help test and mock code within PowerShell. PowerShell isn't only a shell environment. PowerShell is also a scripting language. So, Pester is designed to help create tests for and write PowerShell scripts.
Pester can be useful for a lot of things. For this article, we will be using Pester to test whether the latency for a connection to a device is acceptable. We will also test whether a device or service is responding in an acceptable time frame.
Pester, along with native PowerShell applets, can be used to create a variety of scripts to test network functions and security with. For example, Pester could be used to create a test to ping every IP address on a specific subnet to see if any IP addresses are responding that shouldn't be.
How to Ping in PowerShell
Though pinging equipment on a network with PowerShell is as straightforward as pinging a device with Command Prompt, it's not hard. I'd wager to say that the only thing more difficult about using PowerShell is the number of characters you need to type to send a ping.
So, how do you ping in PowerShell? Use the 'Test-Connection' applet. It's that easy.
Much like you would use 'ping' in CMD, type in 'Test-Connection' followed by '-Ping', '-TargetName', and an IP address to send a ping to that device. The 'Test-Connection' applet will send four ICMP packets to that IP address and log their response times in the PowerShell console. It's that easy.
Of course, 'Test-Connection' has various commands that can be used with it, too. If you need help remembering those commands, type 'Help Test-Connection' into a PowerShell console. That will produce a list of commands that the 'Test-Connection' applet takes as well as the data type it will return.
That is an important thing to remember. PowerShell is different from other shell environments. PowerShell will return values based on various data types while other shell environments log information to the console as strings. If you are writing a script in PowerShell that compares values, you need to remember this. Otherwise, your scripts may not work properly.
There are a few commands that we need to focus on.
The first is the '-TargetName' command. Target name takes its parameters in the form of a string value. So, when you use this command, the IP address you are trying to ping will be read as a string in the 'Test-Connection' applet and not four different octet values. You can also pass an array of IP addresses with '-TargetName' if you want to send a ping to more than one device.
Another command is '-Count'. This command tells 'Test-Connection' how many ICMP packets should be sent to each device you are testing. By default, 'Test-Connection' sends four packets and listens for four responses. You may not want to run four tests, though. In this case, specify the number of times you want 'Test-Connection' to send an ICMP packet with that '-Count' property.
Finally, try to remember the '-TimeoutSeconds' property. By default, 'Test-Connection' will wait several seconds before timing out. If you are positive that your device should respond quicker, you can change the timeout value so that your tests complete faster.
An Overview of Writing Simple Ping Tests with PowerShell [VIDEO]
In this video, Trevor Sullivan covers how to write a simple ping test with the open-source Pester test framework. You will learn how to use the Pester PowerShell module, how to write a practical test within PowerShell, and how to complete a basic ping using the test connection command in PowerShell.
How to Create a Ping Test With Pester in PowerShell
Creating a Pester test to ping devices on a network is easy. Before we dig into that, though, take a look at this GitHub repository.
You will find the example below in the '04-Write a Simple Ping Test' folder.
So, let's dig into this!
Describe -Name "Home Lab" {
Context -Name 'Ping tests' -Fixture {
It -Name 'Should ping default gateway' -Test {
[int](Test-Connection -Ping -ComputerName 10.0.0.1 -Count 1).Latency | Should -BeLessThan 3}
It -Name '10.0.0.77 should not be alive' -Test {
[string](Test-Connection -Ping -ComputerName 10.0.0.77 -Count 1 -TimeoutSeconds 1). Status | Should -Be 'TimeOut'
}
}
}
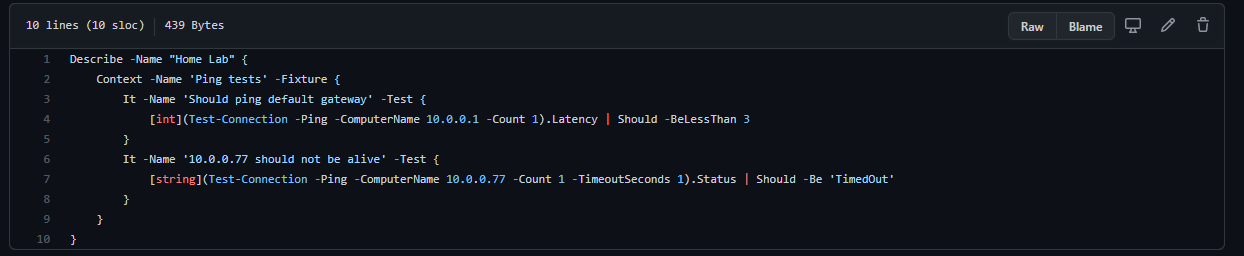
In the above example, we can see that this case test is a simple Describe statement in Pester. We added a Context to this Describe statement as well. This could be useful if you want to perform other tests, like DNS tests, too, or if you want to separate Ping tests per device or subnet.
Inside of each of our contexts, we have two comparison tests. Each comparison test is denoted with the 'Lt' operator. The label for each comparison, or Assertion, is denoted by the '-name' property. When this test case is running, that label will be displayed in the console in PowerShell.
Finally, we have our test to run for each Assertion. First, we are telling that test that any values we get from the test command should be cast as an integer value. Next, we have the test command itself. This is where we use the 'Test-Connection' applet. For this test, we are only trying to compare the latency of the ICMP packet, so we only send one ICMP packet with the '-Count' property. We only need one response. After the test command is run, the value returned by that command is tested to see if it is less than 3. If it is, the test passes. Otherwise, it fails.
The second assertion is basically the same, but it tests whether a connection times out instead.
Wrapping Up
Sending a ping through PowerShell is easy. Instead of using the 'Ping' command as we would in Command Prompt, we would use the 'Test-Connection' applet instead:
Test-Connection -Ping -TargetName 192.168.1.1
The Test-Connection applet collects tons of other useful information, too. For instance, we can get the latency of how long it took a packet to travel round trip or the status code of the ‘Test-Connection’ applet, too.
By understanding what data we can collect with the ‘Test-Connection’ applet, we can use Pester to create automated tests for us. These tests can be used for things like troubleshooting network issues or finding unwanted devices on a network.
If you are the type of person that learns better from watching videos, check out CBT Nugget’s video on the same subject here.
delivered to your inbox.
By submitting this form you agree to receive marketing emails from CBT Nuggets and that you have read, understood and are able to consent to our privacy policy.